Asset Library in the TypeScript SDK
Manage assets using the TypeScript SDK.
The AssetLibrary client in the TypeScript SDK allows create
, list
, get
, and delete
actions of assets. These assets allow integration with Fine-tuning Stable Diffusion.
This guide will walk you through using this API to see a list of our public assets, create your own asset, and use your asset to generate an image.
Requirements
- First, create an OctoAI API token.
- Then, complete TypeScript SDK Installation & Setup..
- If you use the
OCTOAI_TOKEN
environment variable for your token, you can instantiate the client withconst octoai = new OctoAIClient()
or pass the token as a parameter to the constructor.
- If you use the
Overview of AssetLibrary API
Creating a LoRA
You will need a safetensors
file in order to use this example, and in our case one is named origami-paper.safetensors
. I’ll be using a LoRA trained on origami that I can use with the words “origami” and “paper”.
In this example, we will be adding a LoRA then using it to generate an image.
The id
field for the created asset can be used when Getting started with our Media Gen Solution and running inferences.
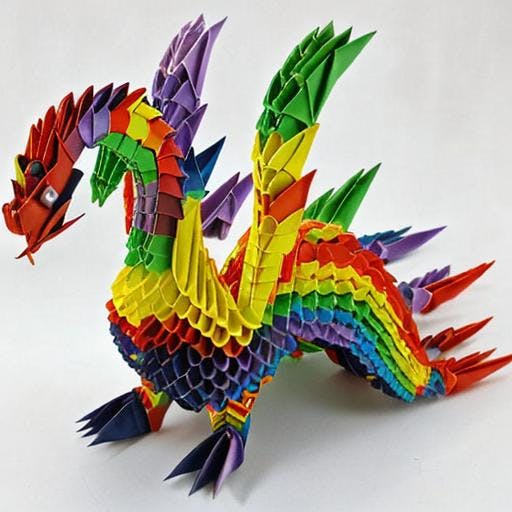
rainbow-origami-tailong-dragon.png generated on ImageGen service using AssetLibrary created LoRA