Asset Library in the Python SDK
Manage assets using the Python SDK.
The AssetLibrary client in the Python SDK allows create, list, get, and delete actions of assets. These assets allow integration with the ImageGenerator Client to generate more customized images.
This guide will walk you through using this API to see a list of our public assets, create your own asset, and use your asset to generate an image.
Requirements
- First, create an OctoAI API token.
- Then, complete Python SDK Installation & Setup..
- If you use the
OCTOAI_TOKEN
envvar for your token, you can instantiate the asset_orch client withasset_library = AssetLibrary()
- If you use the
Overview of AssetLibrary API
Creating a LoRA and Generating an Image
You will need a safetensors
file in order to use this example, and in our case one is named origami-paper.safetensors
. I’ll be using a lora trained on origami that I can use with the words “origami” and “paper”.
In this example, we will be adding a LoRA then using it to generate an image. You can also add checkpoints
, vae
, and textual inversions
.
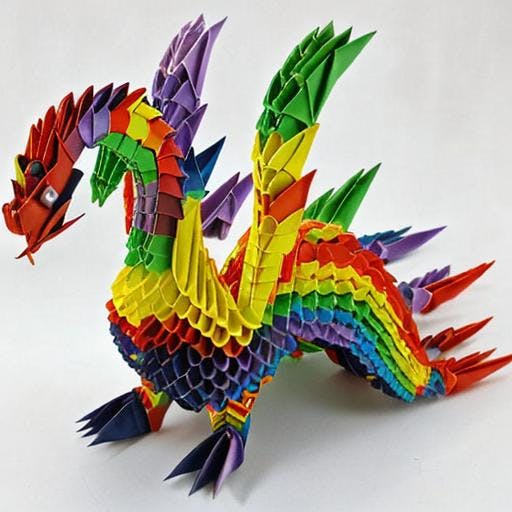
rainbow-origami-tailong-dragon.png
Creating File Assets from a Folder of Images
Let’s say you have a folder of images assets you would like to upload for using the FineTuning service. You can do so using the below code snippet to get all the files in your folder named images
, and then splitting on the .
to get your file_format extension (jpg
, jpeg
, or png
), and use the file name as the asset name.
In this example, there is a directory named images
that contains files with a _, -, and alphanumeric file names, jpg, jpeg, or png suffixes. In this example, the folder contains the following:
You can then use octoai.asset_library.list()
to see the assets have been created and uploaded and a result that looks something like:
In this example, an already existing lora created in the previous example also exists. The lora has uploaded however will likely need a few more seconds before being ready for use.